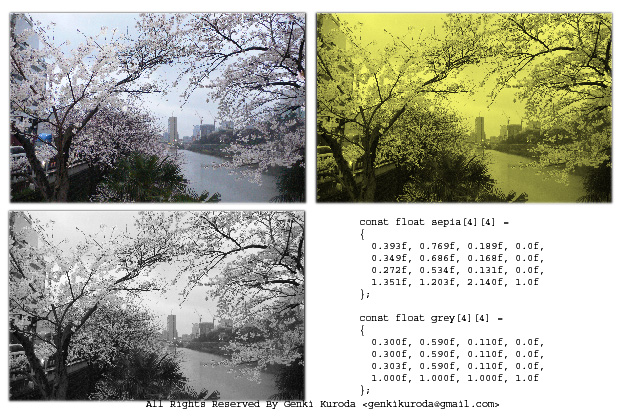
This diagram shows one original image at top left and two images after color filter.
The right top one is post processed by Sepia color filter at Fragment Shader.
The left bottom one is post processed by Grey color filter at Fragment Shader, which may be not clear but it is mono tone color.
I think simple color filters are easiest thing to start with.
Also, I made the fragment shader to be simplest.
Basically the DirectX program send a 4×4 color filter matrix. It is usable for color filter which take a pixel as input and modify the color using the given pixel color.
For example,
Sepia Color Filter is declared as follows:
const float sepia[4][4] =
{
0.393f, 0.769f, 0.189f, 0.0f,
0.349f, 0.686f, 0.168f, 0.0f,
0.272f, 0.534f, 0.131f, 0.0f,
1.351f, 1.203f, 2.140f, 1.0f
};
By using this 4×4 matrix, I implemented in the fragment shader as follows:
Pseudo-code
pixel.r = vector4f(pixel) * vector4f(0.393f, 0.769f, 0.189f, 0.0f,)
pixel.g = vector4f(pixel) * vector4f(0.349f, 0.686f, 0.168f, 0.0f)
pixel.b = vector4f(pixel) * vector4f(0.393f, 0.769f, 0.189f, 0.0f,)
pixel = pixel / vector4f(1.351f, 1.203f, 2.140f, 1.0f)
[Fragment Shader in Cg]
pixel main( fragment IN,
uniform sampler2D testTexture,
uniform float4 colorFilter[4])
{
pixel texture, OUT;
//Load Texture Color
texture.color = tex2D( testTexture, IN.texcoord0 );
//Color Filer
float4 result;
result.r = sum(texture.color * colorFilter[0]);
result.g = sum(texture.color * colorFilter[1]);
result.b = sum(texture.color * colorFilter[2]);
result.a = 1.0;
result /= colorFilter[3];
OUT.color = float4(result.r, result.g, result.b, result.a);
return OUT;
}